Link Adaptation
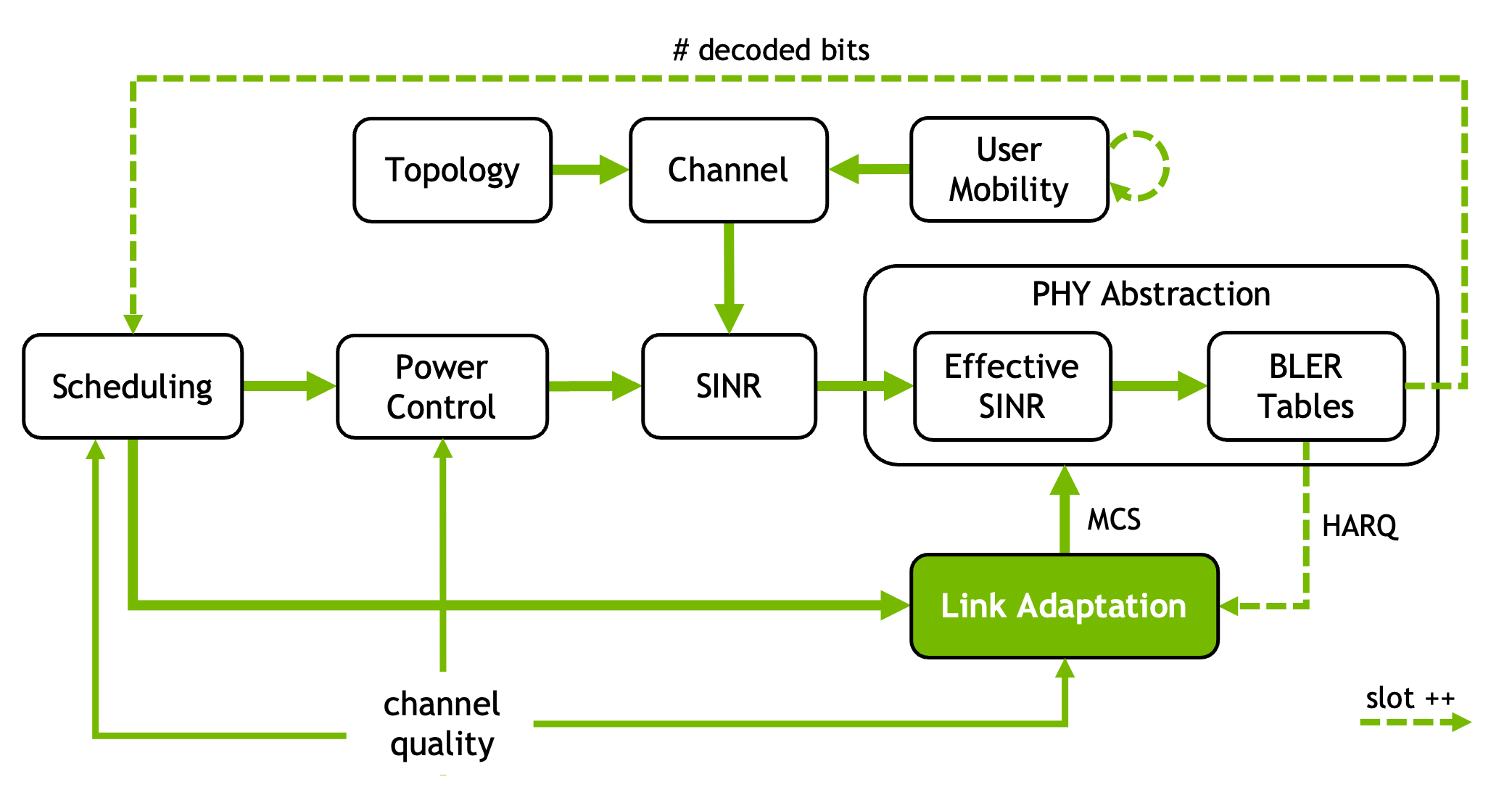
Link adaptation (LA) optimizes the performance of a single wireless link by dynamically adjusting transmission parameters to match time-varying channel conditions.
The objective is to maximize the achieved throughput while maintaining the transport block error rate (TBLER) sufficiently low. Typically, this is simplified to maintaining the TBLER close to a predefined target, designed to balance throughput and latency.
The main challenge lies in handling noisy and sparse SINR feedback, which is used to estimate the channel quality.
For a usage example of link adaptation in Sionna, we refer to the Link Adaptation notebook.
- class sionna.sys.InnerLoopLinkAdaptation(phy_abstraction, bler_target=0.1, fill_mcs_value=0)[source]
Class for inner loop link adaptation (ILLA). It computes the highest available modulation and coding scheme (MCS) whose associated transport block error rate (TBLER) does not exceed the specified
bler_target
:where
is the effective SINR value provided as input. If no such MCS exists, the lowest available MCS index is returned. If a user is not scheduled,fill_mcs_value
is returned.- Parameters:
phy_abstraction (
PHYAbstraction
) – An instance ofPHYAbstraction
bler_target (float (default: 0.1)) – BLER target
fill_mcs_value (int (default: 0)) – MCS value assigned to non-scheduled users
- Input:
sinr ([…, num_ofdm_symbols, num_subcarriers, num_ut, num_streams_per_ut], tf.float | None (default)) – SINR for each OFDM symbol, subcarrier, user and stream. If None, then
sinr_eff
andnum_allocated_re
are both required.sinr_eff ([…, num_ut], tf.float | None (default)) – Estimated effective SINR for each user. If None, then
sinr
is required.num_allocated_re ([…, num_ut], tf.int32 | None (default)) – Number of allocated resources in a slot, computed across OFDM symbols, subcarriers and streams, for each user. If None, then
sinr
is required.mcs_table_index ([…, num_ut], tf.int32 | int (default: 1)) – MCS table index for each user. For further details, refer to the Note.
mcs_category ([…, num_ut], tf.int32 | int (default: 0)) – MCS table category for each user. For further details, refer to the Note.
return_lowest_available_mcs (bool (default: False)) – If True, the lowest MCS available in
phy_abstraction
BLER tables is returned for each user. Only used for internal purposes.
- Output:
mcs_index ([…, num_ut]) – Highest available MCS whose BLER does not exceed the target, or the lowest available MCS if no such MCS exists, for each user
Example
from sionna.sys import PHYAbstraction, InnerLoopLinkAdaptation bler_target = 0.1 # Initialize the PHY abstraction object phy_abs = PHYAbstraction() # Initialize the ILLA object illa = InnerLoopLinkAdaptation(phy_abs, bler_target=0.1) # Effective SINR for each user sinr_eff = tf.Variable([0.1, 10, 100]) # N. allocated resource elements for each user num_allocated_re = tf.Variable([20, 30, 30]) # Compute the MCS index for each user mcs_index = illa(sinr_eff=sinr_eff, num_allocated_re=num_allocated_re, mcs_table_index=1, mcs_category=0) print('Selected MCS index =', mcs_index.numpy()) % Selected MCS index = [ 3 16 27]
- property bler_target
Get/set the BLER target for each user
- Type:
tf.float
- class sionna.sys.OuterLoopLinkAdaptation(phy_abstraction, num_ut, bler_target=0.1, delta_up=1.0, batch_size=None, sinr_eff_init=1.0, sinr_eff_init_fill=1.0, offset_min=-20.0, offset_max=20.0)[source]
Class for outer-loop link adaptation (OLLA). The modulation and coding scheme (MCS) index for a user is determined as the highest index whose corresponding transport block error rate (TBLER) remains below the specified
bler_target
. The SINR value used for TBLER computation is given by the last effective SINR feedback, [dB], reduced by an offset value, :The value of
is adjusted depending on the HARQ feedback [Pedersen05]:where the relationship between
and is given by [Sampath97]:- Parameters:
phy_abstraction (
PHYAbstraction
) – An instance ofPHYAbstraction
num_ut (int) – Number of user terminals
bler_target (float (default: 0.1)) – BLER target value, within 0 and 1
delta_up (float (default: 1.)) – Increment applied to the SINR offset [dB] when a NACK is received for a user
batch_size (list | int | None (default)) – Batch size or shape. It accounts for multiple users for which link adaptation is performed simultaneously. If None, the batch size is set to [].
sinr_eff_init ([…, num_ut], tf.float | float (default: 1.)) – Initial value of effective SINR for each user. Non-positive values are treated as missing and replaced by
sinr_eff_init_fill
. If float, the same value is assigned to all users.sinr_eff_init_fill (float (default: 1.)) – Value replacing non-positive
sinr_eff_init
valuesoffset_min (float (default: -20.)) – Minimum SINR [dB] offset value
offset_max (float (default: 20.)) – Maximum SINR [dB] offset value
- Input:
num_allocated_re ([…, num_ut], tf.int32) – Number of allocated resources in the upcoming slot, computed across OFDM symbols, subcarriers and streams, for each user
harq_feedback ([…, num_ut], -1 | 0 | 1) – If 0 (1, resp.), then a NACK (ACK, resp.) is received. If -1, feedback is missing.
sinr_eff ([…, num_ut], tf.float | None (default)) – Estimated effective SINR for each user. Non-positive values are treated as missing.
mcs_table_index ([…, num_ut], tf.int32 | int (default: 1)) – MCS table index for each user. For further details, refer to the Note.
mcs_category ([…, num_ut], tf.int32 | int (default: 0)) – MCS table category for each user. For further details, refer to the Note.
- Output:
mcs_index ([…, num_ut]) – Selected MCS index for each user
- property bler_target
Get/set the BLER target for each user
- Type:
tf.float
- property delta_down
Decrement applied to the SINR offset when an ACK is received for a user. Computed as
delta_up * bler_target / (1 - bler_target)
.- Type:
float (read-only)
- property delta_up
Get/set the increment applied to the SINR offset when a NACK is received for a user
- Type:
float
- property offset
Effective SINR [dB] offset for each user
- Type:
[…, num_ut], tf.float (read-only)
- property offset_max
Get/set the maximum
offset
value- Type:
tf.float
- property offset_min
Get/set the minimum
offset
value- Type:
tf.float
- reset(sinr_eff_init=1.0, sinr_eff_init_fill=0.1)[source]
Resets the values of
sinr_eff_db_last
andoffset
- property sinr_eff_db_last
Get/set the last observed effective SINR [dB] value for each user
- Type:
[…, num_ut], tf.float
Note
The concepts of MCS table index, category, code block and transport blocks are inspired by but not necessarily tied to 3GPP standards. It is assumed that:
Each MCS category has multiple table indices, each defining the mapping between MCS indices and their corresponding modulation orders and coding rates. Such relationships are defined by
sinr_effective_fun
;The transport block, which serves as main data unit, is divided into multiple code blocks. The number and size of these code blocks are computed by
transport_block_fun
.
Yet, if neither sinr_effective_fun
nor transport_block_fun
is provided,
this class aligns with 3GPP TS 38.214 ([3GPP38214]),
specifically Section 5.1.3 (PUSCH) and Section 6.1.4 (PDSCH).
In this case, the MCS category refers to PDSCH (category = 1) and PUSCH (0).
Valid table indices are {1, 2} for PUSCH and {1, 2, 3, 4} for PDSCH.
For more information, refer to TBConfig
.
- References:
- [Pedersen05]
K. I. Pedersen, G. Monghal, I. Z. Kovacs, T. E. Kolding, A. Pokhariyal, F. Frederiksen, P. Mogensen. “Frequency domain scheduling for OFDMA with limited and noisy channel feedback,” IEEE Vehicular Technology Conference (VTC), pp. 1792-1796, Sep. 2007.
[Sampath97]A. Sampath K. P. Sarath, J. M. Holtzman. “On setting reverse link target SIR in a CDMA system.” 1997 IEEE 47th Vehicular Technology Conference. Technology in Motion. Vol. 2. IEEE, 1997.